Dynamic Programming
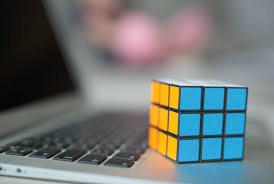
What is dynamic programming? It is a way of making your algorithm more efficient by storing some of the intermediate results and it works really well when your algorithm has a lot of repetitive computations. So that you don't have to repeat those computations over and over again. I'm gonna give you a concrete example of how it works with Fibonacci Sequence (1, 1, 2, 3, 5, 8, ....). Let see how we can solve the problem using dynamic programming. There are typically three steps you can take: 1. Recursion. 2. Store (Memoize). 3. Bottom-up. Recursion solution public static int fibSeq (int n) { // this function return the value of Fibonacci Sequence at n position if(n ==1 || n==2) return 1; else return (fibSeq(n-1) + fibSeq(n-2)); } // Let call this function return value 5 fastly fibSeq(5); // Return 6765 value pretty quickly on my computer fibSeq(20); // Return value 9227