Create Visualforce page using Standard Controller and Extensions in Salesforce
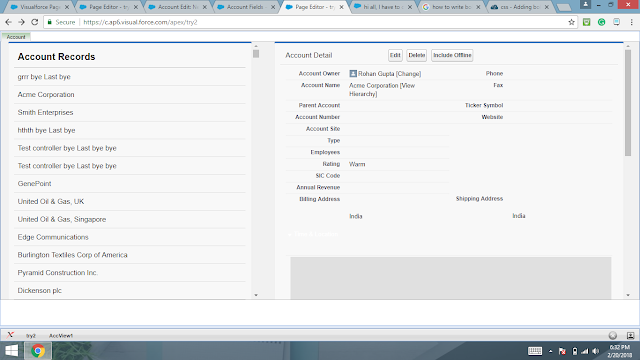
In this post, I am going to tell you how to use Standard Object and Extensions in a visualforce page. Task: When you click on an Account's record, it will display the fields data of selected record. First of all, I am going to design a visualforce page look like this. Function: We create a tab whose name is Account . It has two part one is Account Records and another is Account Detail as shown in the figure. In Account Records section, we display all records of the Account object. In Account Details section, we display the details of the selected Account's record. Source code: Visualforce page <apex:page showHeader="false" sidebar="false" standardController="Account" extensions="AccView1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"/> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.